This is a continuation of the API series. Part one about the history of APIs is here.
The process of creating high-quality APIs is complex and requires careful planning. A service or application consists of multiple APIs which are then aligned with back-end operations.
This information is presented using Representational State Transfer, or REST API concepts that are the most common APIs on the web. And an API is a contract between the application provider and the consumer. An intermediary between a service and it’s users or clients. Here, clients refers to services that send requests to an API.
As mentioned in the hitory of APIs, APIs can be public facing or private. An example of public APIs is a robot taxi service. This service will offer robotic transportation to dfestinations of their choosing. The service could allow developers, such as food delivery services and grocery stores, to use their APIs to support their business.
Continuing with a robot taxi service, their APIs could include creating an account, ordering a taxi, and scheduling a recurring transport service. From a REST(ful) perspective, creating an account and ordering a taxi is a POST operation, rescheduling the pickup date and/or time is an UPDATE operation and reviewing a scheduled pickup is a GET operation.
Another way of describing APIs are as a set of operations within an application or service. APIs are an intermediate representation of how a service’s back-end operations are organized.
Create An API
Creating a service or application’s APIs requires detailed planning and preparation. It involves considering what operations the service will provide and organizing them into logical buckets. Requests that create new records must be distinguished from those that update, or delete them. Even with the space of requests that create new records, depending on the range of new record types, there may need to be one POST operation defined for each one. A good example is the API to create a checking account versus an investing account may be different enough to require that their POST operations are unique.
It involves coding back-end operations, data storage and manipulation, and sometimes a front-end interface. A successful set of APIs are extensively planned and tested end-to-end. Once the APIs are developed, with or without a front-end, they can remain private or be shared publicly.
Before sharing, APIs should be documented such that engineers and other services can use them. The documentation should contain detailed descriptions of each endpoint, its operations and how they work. Ideally, the documentation will include a rudimentary interface for developers to test them. A popular option to test an API is with cURL but it can also be a simple web interface.
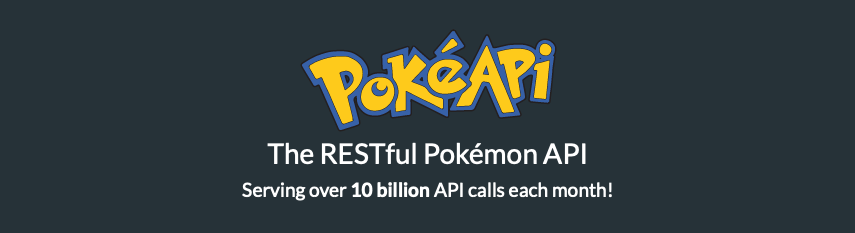
For example, the PokéAPI documentation includes a simple interface to test their APIs.
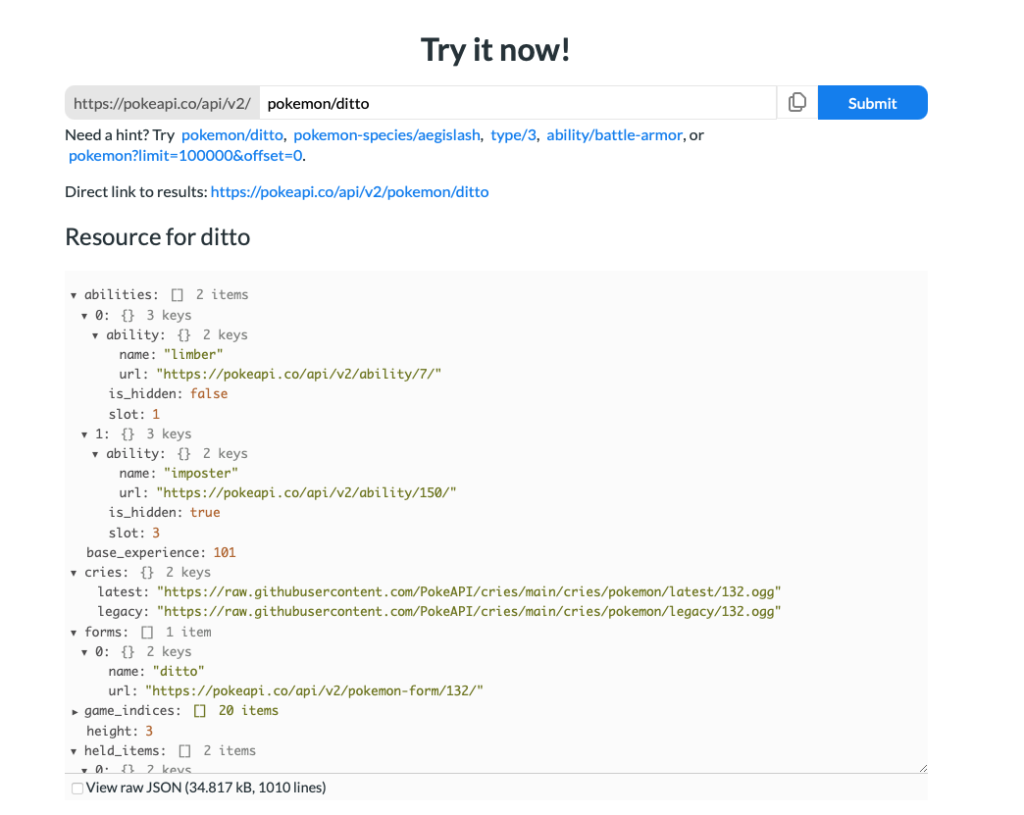
Finally, your APIs can be published in a directory such as Postman, or an independent website. There isn’t one centralized directory of APIs but a quick topic-focused API google search will return results.
It Begins With An Idea
APIs always begin with a big idea or concept to create a solution to an existing problem. An idea that is then defined in a detailed design document and is organized and developed into service operations.
The new application begins with a high-level description of its purpose and features. It states the problem it’s going to solve. Before the project is created and work begins, a feasibility analysis that includes market research is done to see if the proposed solution is viable. Even if there are existing solutions on the market, your service may introduce features and ideas that are different enought to hold a market niche.
A roadmap is then developed that defines the features-at-launch and subsequent feature releases. The initial features are considered to be a minimally viable product (MVP). With a roadmap, it will be clear which features will be minimally viable for the first release and which ones will be in subsequent ones in a given timeframe. After all, development of a service or application can’t go on forever .
In the early stages of a service’s design, even the MVP feature wishlist at the start may have to be scaled back to meet the production deadline. Not all features will be feasible at launch and not all will be implemented due to time and other resource constraints.
Initial Design Document
Once the MVP is defined, a design document is written. A design document will function as a technical guide for how the MVP will be developed and tested. It is intended to provide the solutions to deliver the MVP based on the service’s roadmap. It will create the framework that will be used by developers to deliver the service.
The design document should address these main areas:
- Available operations
- Data requirements
- Existing frameworks to leverage
- Testing and validation
- Security considerations
- Front-end UI development (if any)
Each of these main areas require detailed planning and consideration to orchestrate them under the specified MVP endpoints, or APIs. It will require detailed analysis of existing code to identify what has to be created and what can be reused. It also needs input from project stakeholders and supporters.
One important development step is to research and leverage existing frameworks that can be used to speed up development. The use of existing APIs will avoid having to write all of the code from scratch. Leveraging these resources is an important part of modern software development.
The remaining areas to include in the document will also require further detailed analysis from the perspective of the whole service. For example, data management can’t be considered separately from security considerations and existing frameworks. For each area there should be implementation details and examples. It must specify how the operations will be organized and integrated into a coherent service. This information is critical for engineers to develop the product and for stakeholders to maintain their support of the project.
Design Review
After the design document is written, it is presented to the team and stakeholders for review and feedback. The review should include a subject matter expert. The design review is intended to refine and improve the implementation. It ensures that development will follow several key software engineering principles such as:
- Modularity: software decomposed into independent, reusable modules with a specific purpose
- Abstraction: hide complex implementation details and only expose necessary information
- Encapsulation: bundle data and operations within a single unit and protecting data from external access
- DRY: stands for don’t repeat yourself and is related to modularity by avoiding redundant code
- CI/CD: A method for automatically integrating and deploying software releases Each member on the team may have specialized knowlegdge or insight they can contribute to improving the design and ultimate delivery of the service.
The design reviewers will analyze each operation, or endpoint to ensure that it will be secure, reliable, perform as expected and manage its data securely and efficiently. Reviewers will provide feedback or inquiries, prompting the designer to address all feedback before the next review session.
Design reviews are iterative in that with each review round results in actionable feedback that the design document writer will implement before conducting another round of reviews. The service design is finalized when the key stakeholders give their final approval for implementation to begin. Sometimes approval is an informal communication.
Implementation
Once the team has reviewed and finalized the service’s design, implementation can begin. The logic for each operation, including data management will be implemented. If it’s a RESTful service, it’s operations will align with operations that GET, POST, PUT, and DELETE.
Development is done in sprints. The design implementation and plan is further broken down into pieces of work that can be done typically within one to two weeks. This way the project can deliver incremental progress while development continues. It’s a good morale boost for the development team to see their work making a difference, and for stakeholders to maintain their support.
Along the way, there will be extensive testing such as unit, integration and load testing. Unit testing will verify that the functions within an operation work as expected. Integration testing will ensure that each operation works end-to-end as expected. Load testing will simulate traffic or requests to the service. In load testing, the goal is to find the service’s upper limits based on the service’s hardware, network and data. Testing during development is a crucial process throughout the services initial launch and beyond.
Once the operations are developed and tested, the next steps are to configure the operatons as API endpoints. These endpoints will be used by clients and developers. As with operation development, APIs also must be tested, test API endpoint access, write API documentation and develop the front-end.
Conclusion
Creating high-quality APIs, which function as critical intermediaries or contracts connecting a service with its clients, demands meticulous planning and preparation, starting with a core idea to address a problem. This complex process involves carefully organizing back-end operations, often adopting RESTful principles with operations like GET and POST, detailing the system within a comprehensive design document that covers key areas such as data management, security, and leveraging existing frameworks. The design is then subjected to thorough, iterative review by stakeholders and subject matter experts to refine it before implementation begins in phases or sprints, coupled with continuous, rigorous testing—spanning unit, integration, and load testing—to ensure quality and performance prior to final documentation and potential sharing, whether privately or publicly.