This post will demonstrate how to use docopt in Python code to create CLI documentation. Docopt is a tool for creating and presenting documentation for command-line functions and scripts. Finally, we will demonstrate what the help documentation looks like at the command line.
The example code we’ll use is a reverse string function that does not provide any help information for users to understand. The function itself is simple: It takes a string as input and returns it in reverse order. When the function is invoked, a user is prompted to type a string followed by the enter key. The reversed string will be returned to the user.
The implementation of docopt will be broken down into four steps. Step one will install the tool. Step two will show how to import docopt for Python. Step three will add the help documentation as a docstring. Step five will implement docopt in the main function. At the end of this post, you’ll see the completed implementation.
Step One
The first step for Python is to install docopt for Python at the command line with the command below. We’ll use pip to install Docopt, Python’s standard package manager.
pip install docopt
Step Two
The second step is to open the reverse_string_with_docopt.py
file and add an import statement above the function.
from docopt import docopt
Step Three
The third step is to add the following Python docstring to the beginning of the file, before the import statement. The docstring contains help sections for usage and options. The usage section defines how to invoke the function with arguments and options, while the options section specifies the available long and short options.
"""
Reverse String
Reverses any input string.
Usage:
reverse_string.py [reverse]
reverse_string.py -h | --help
reverse_string.py -v | --verbose
Options:
-h --help Show this
-v --verbose Verbose mode
-q --quiet Quiet mode
"""
In the usage section, ‘reverse’ will invoke the function. The options ‘-h’, ‘-v’, and ‘-q’ will return the help information in various formats. The first two options will return the contents of the docstring. The third option, ‘-q’, will return the current invocation setting for all options.
Step Four
The fourth step is to add a main
function to specify how the function and help documentation will be invoked. The arguments
variable will receive the arguments passed from the command prompt, and the if/else block will execute the code based on the argument provided.
The if block executes the reverse string function when ‘reverse’ is passed in as the argument. This prompts the user to enter a string and returns its reverse. The else block displays help or exits the running code.
if __name__ == '__main__':
arguments = docopt(__doc__)
if arguments['reverse']:
string_to_reverse = input('Enter the string to be reversed: ')
reverse(string_to_reverse)
else:
print(arguments)
The argument
is provided at the command line immediately after the Python script’s name. The __doc__
parameter could be a docstring or some other string that contains a help message to be parsed.
The Complete Script
The complete Python script is provided below and can be downloaded here on Github. The help section is at the top, followed by an import statement, the reverse function, and the main function.
"""
Reverse String Function
Reverses any input string.
Usage:
reverse_string.py [reverse]
reverse_string.py -h | --help
reverse_string.py -v | --verbose
Options:
-h --help show this
-v --verbose verbose mode
-q --quiet quiet mode
"""
from docopt import docopt
def reverse(string_to_reverse):
reversed_str = string_to_reverse[::-1]
print(reversed_str)
if __name__ == '__main__':
arguments = docopt(__doc__)
if arguments['reverse']:
string_to_reverse = input('Enter the string to be reversed: ')
reverse(string_to_reverse)
else:
print(arguments)
Demo Usage
Use the text below to execute the code type at the command line. If the current working directory isn’t where the file is located, navigate to it first before using this string. Alternatively, you can use the complete path to the file. For example, the file path on a Mac could be ./My Documents/Python\reverse_string_with_docopt.py
, where the period (‘.’) represents your home folder.
The command will invoke the function without displaying any help documentation.
python reverse_string_with_docopt.py reverse
You will be prompted to enter the string to be reversed.
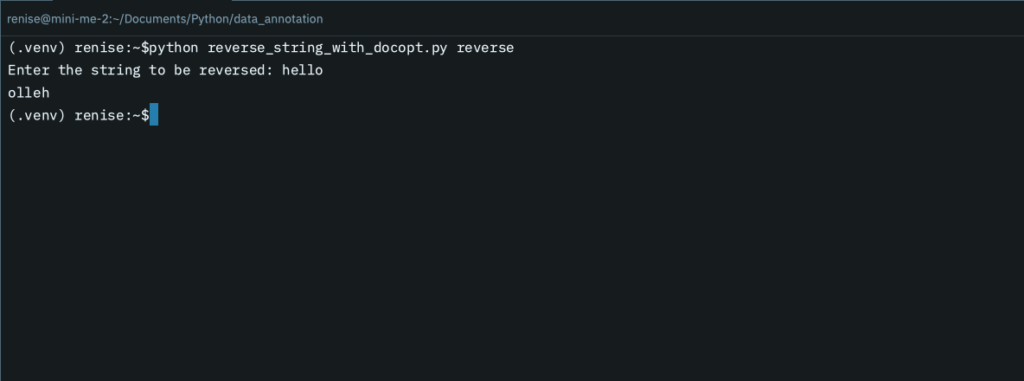
To view the reverse function’s help documentation, use the options -h
or —help
.
python reverse_string_with_docopt.py -h
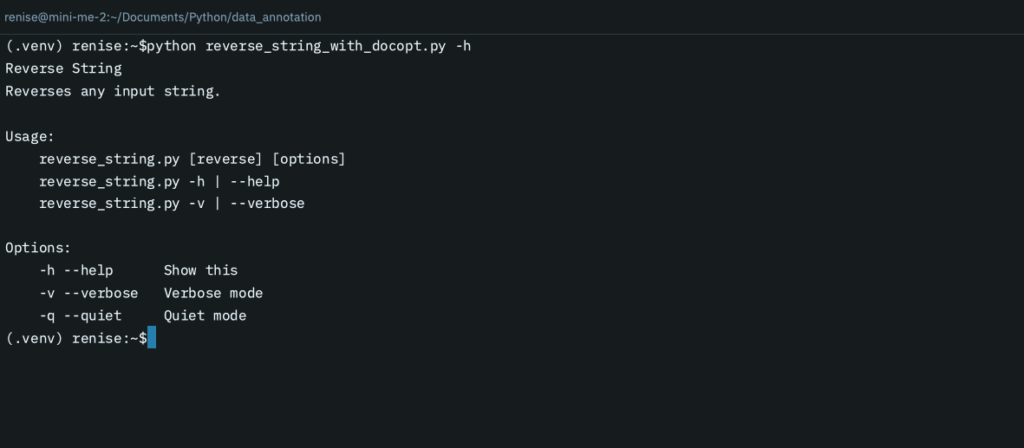
The result will display the help documentation added to the top of the file, as seen above. When -q
is invoked, it shows which option was used.
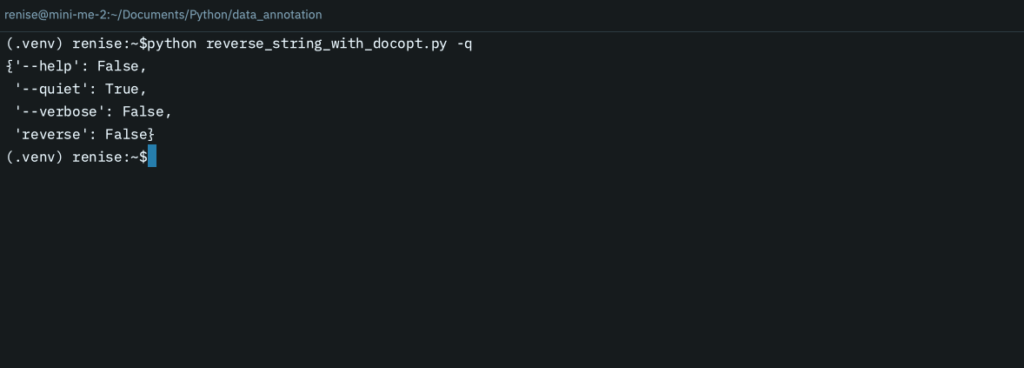
Conclusion
The provided example is simple, but the principles are the same regardless of the lines of code. It shows how to install and implement docopt with Python. There are other variants for C++, Go, and Ruby, to name a few. Go to Github to view all variants. It’s important to know that CLI documentation isn’t a replacement for standalone documentation such as a webpage or PDF.